Custom Post Type Snippets to make you smile
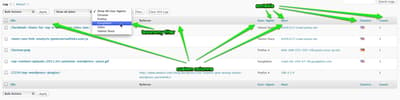
So it’s friday, I’ve been coding all day and I thought I’d share some of the cool snippets I’ve come across and/or developed today. I’ve mostly been working with Custom Post Types and Taxonomies, so let me share some of that goodness. Let’s geek out in a bit, but first let me show you why this is cool, be sure to click the image, so you can see which functionality I’ve added to the otherwise boring custom posts overview screen:
Add columns to the overview page for a Custom Post Type
So you’ll want to add some columns to your post type’s overview page, or remove some. Don’t forget to replace <CPT> with your own custom post type in all these examples:
[code lang=”php”]// Change the columns for the edit CPT screen
function change_columns( $cols ) {
$cols = array(
‘cb’ => ‘<input type="checkbox" />’,
‘url’ => __( ‘URL’, ‘trans’ ),
‘referrer’ => __( ‘Referrer’, ‘trans’ ),
‘host’ => __( ‘Host’, ‘trans’ ),
);
return $cols;
}
add_filter( "manage_<CPT>_posts_columns", "change_columns" );[/code]
Be sure to always leave the cb
column in there or your mass edit / delete functionality will not work.
Give these new columns some content
Now let’s fill these new columns with some content from the custom post type:
[code lang=”php”]function custom_columns( $column, $post_id ) {
switch ( $column ) {
case "url":
$url = get_post_meta( $post_id, ‘url’, true);
echo ‘<a href="’ . $url . ‘">’ . $url. ‘</a>’;
break;
case "referrer":
$refer = get_post_meta( $post_id, ‘referrer’, true);
echo ‘<a href="’ . $refer . ‘">’ . $refer. ‘</a>’;
break;
case "host":
echo get_post_meta( $post_id, ‘host’, true);
break;
}
}
add_action( "manage_posts_custom_column", "custom_columns", 10, 2 );[/code]
Make these new columns sortable
Now this extra info is cool, I bet you want to sort by it, that’s as simple as this:
[code lang=”php”]// Make these columns sortable
function sortable_columns() {
return array(
‘url’ => ‘url’,
‘referrer’ => ‘referrer’,
‘host’ => ‘host’
);
}
add_filter( "manage_edit-<CPT>_sortable_columns", "sortable_columns" );[/code]
Filter Custom Posts by Custom Taxonomy
Ok so far this is all fairly simple. Now let’s go a bit more advanced. Let’s say you have a custom taxonomy attached to that custom post type and you want to show a filter for that custom taxonomy on the custom post types overview page, just like you have a categories drop down on the posts overview page. This code was taken (though slightly modified) from this thread.
Let’s first add that dropdown / select box to the interface:
[code lang=”php”]// Filter the request to just give posts for the given taxonomy, if applicable.
function taxonomy_filter_restrict_manage_posts() {
global $typenow;
// If you only want this to work for your specific post type,
// check for that $type here and then return.
// This function, if unmodified, will add the dropdown for each
// post type / taxonomy combination.
$post_types = get_post_types( array( ‘_builtin’ => false ) );
if ( in_array( $typenow, $post_types ) ) {
$filters = get_object_taxonomies( $typenow );
foreach ( $filters as $tax_slug ) {
$tax_obj = get_taxonomy( $tax_slug );
wp_dropdown_categories( array(
‘show_option_all’ => __(‘Show All ‘.$tax_obj->label ),
‘taxonomy’ => $tax_slug,
‘name’ => $tax_obj->name,
‘orderby’ => ‘name’,
‘selected’ => $_GET[$tax_slug],
‘hierarchical’ => $tax_obj->hierarchical,
‘show_count’ => false,
‘hide_empty’ => true
) );
}
}
}
add_action( ‘restrict_manage_posts’, ‘taxonomy_filter_restrict_manage_posts’ );[/code]
And then, we add a filter to the query so the dropdown will actually work:
[code lang=”php”]function taxonomy_filter_post_type_request( $query ) {
global $pagenow, $typenow;
if ( ‘edit.php’ == $pagenow ) {
$filters = get_object_taxonomies( $typenow );
foreach ( $filters as $tax_slug ) {
$var = &$query->query_vars[$tax_slug];
if ( isset( $var ) ) {
$term = get_term_by( ‘id’, $var, $tax_slug );
$var = $term->slug;
}
}
}
}
add_filter( ‘parse_query’, ‘taxonomy_filter_post_type_request’ );[/code]
Note that for these last two snippets to work, query_var
must have been set to true when registering the custom taxonomy, otherwise this’ll never work.
Bonus: Add Custom Post Type to feed
This one came courtesy of Remkus de Vries a while back and was helpful today, adding a custom post type to your site’s main feed, don’t forget to replace <CPT> with your own custom post type:
[code lang=”php”]// Add a Custom Post Type to a feed
function add_cpt_to_feed( $qv ) {
if ( isset($qv[‘feed’]) && !isset($qv[‘post_type’]) )
$qv[‘post_type’] = array(‘post’, ‘<CPT>’);
return $qv;
}
add_filter( ‘request’, ‘add_cpt_to_feed’ );[/code]
Coming up next!
-
Pride Amsterdam 2025
August 02, 2025 Team Yoast is at Attending Pride Amsterdam 2025! Click through to see who will be there, what we will do, and more! See where you can find us next » -
The SEO Update by Yoast - June 2025 Edition
24 June 2025 Expert analysis of the latest SEO & AI news developments with Carolyn Shelby and Alex Moss. Join our upcoming update! 📺️ All Yoast SEO webinars »
Discussion (20)